2022. 9. 20. 23:14ㆍ웹 개념
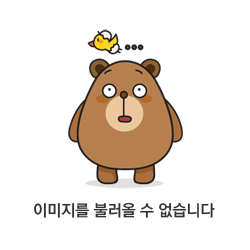
1. 우선 express.js를 이용하여 간단하게 백앤드 서버를 구현해보자!
express를 가져오고 가져온 express함수를 app엠 담고, 포트번호는 7777로 설정.
<app.js>
// express libaray fetch
const express = require("express");
// 함수 담기
const app = express();
const PORT = 7777;
2. listen을 사용해서 해당 포트 번호로 서버를 엽니다. 그리고 get을 이용해서 '/' 위치의 index.html을 서버에서 가져오는 코드를 작성합니다.
// __dirname 현재 경로를 의미한다.
app.get('/', (req, res) => {
res.render("index.html");
})
app.listen(PORT, () => {
console.log("server open: " + PORT);
})
3. res로 응답을 받아서 render를 이용해서 index.html를 랜더링하기로 했으니 우선 index.html을 만들어 봅시다~
<index.html>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>index page</title>
<script src="https://code.jquery.com/jquery-3.6.1.js" integrity="sha256-3zlB5s2uwoUzrXK3BT7AX3FyvojsraNFxCc2vC/7pNI=" crossorigin="anonymous"></script>
</head>
<body>
<h1>index page</h1>
<a href="/user/login">로그인</a>
<a href="/user/signUp">회원 가입</a>
</body>
</html>
4. 이렇게 완성하고 사이트를 열어보면~? 안열릴 겁니다.
index.html을 views폴더 안에 넣어놨는데. express는 그게 views있는지 모릅니다 때문에 app.js에서 이부분을 고려한 코드를 넣어야 합니다.
또한 html이 랜더링 될 때 ejs엔진을 이용해야 합니다.
// view 경로 설정
app.set('views', __dirname + "/views")
// npm i ejs
// 서버가 HTML 렌더링을 할 때, EJS엔진을 사용하도록 설정합니다.
app.set('view engine', 'ejs')
app.engine('html', require('ejs').renderFile);
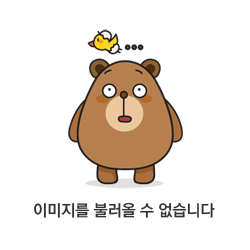
짜잔 ~ 다음과 같은 페이지를 보실 수 있습니다.
5. 이제 ajax를 이용하여 회원가입 로그인 데이터를 받아서 로그인 기능과 회원 가입 기능을 해줘 봅시다!!
회원 가입부분 부터 시작합니다. 위의 html 코드를 보면 a태그로 /user/login, /user/signUp 주소로 가는게 보이시죠?
이제 이걸 할려면 app.js를 조금 변형해야 합니다.
<app.js>
// http://localhost:7777/user/ => 이 경로를 통해 userRouter에 접근
const userRouter = require('./routes/user');
ajax를 이용해서 데이터를 받으면 body로 데이터를 받을 수 있습니다.
이때 해당 데이터를 json으로 인코딩해야 읽을 수 있습니다.
// body에 들어오는걸 json 인코딩
app.use(express.json());
// body에 문자열로 url이 들어오는걸 인코딩
app.use(express.urlencoded({ extended: false }));
그리고 아까 userRouter의 경로를 이용.
app.use("/user", userRouter) // /user 경로로 가면 userRouter를 이용해! 라는 뜻.
이렇게 추가 코드를 작성해야 합니다.
결론적으로 전체 코드는 아래와 같이 됩니다.
<app.js>
// express libaray fetch
const express = require("express");
// 함수 담기
const app = express();
const PORT = 7777;
// http://localhost:7777/user/ => 이 경로를 통해 userRouter에 접근
const userRouter = require('./routes/user');
// body에 들어오는걸 json 인코딩
app.use(express.json());
// body에 문자열로 url이 들어오는걸 인코딩
app.use(express.urlencoded({ extended: false }));
// view 경로 설정
app.set('views', __dirname + "/views")
// npm i ejs
// 서버가 HTML 렌더링을 할 때, EJS엔진을 사용하도록 설정합니다.
app.set('view engine', 'ejs')
app.engine('html', require('ejs').renderFile);
app.use("/user", userRouter) // /user 경로로 가면 userRouter를 이용해! 라는 뜻.
// __dirname 현재 경로를 의미한다.
app.get('/', (req, res) => {
res.render("index.html");
})
app.listen(PORT, () => {
console.log("server open: " + PORT);
})
6. 그럼 이제 views폴더 안에 보여질 login, sigonUp HTML파일을 만들어봅시다.
<login.html>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>login page</title>
<script
src="https://code.jquery.com/jquery-3.6.1.js"
integrity="sha256-3zlB5s2uwoUzrXK3BT7AX3FyvojsraNFxCc2vC/7pNI="
crossorigin="anonymous"
></script>
</head>
<body>
<h1>login page</h1>
<a href="/">홈</a>
<a href="/user/signUp">회원 가입</a>
<br />
<br />
<form id="loginForm">
<input
type="text"
id="id"
name="id"
placeholder="please enter a your id"
/>
<br />
<input
type="password"
id="pwd"
name="pwd"
placeholder="please enter a your password"
/>
<input type="button" value="login" onclick="sendData()" />
</form>
</body>
</html>
<signUp.html>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<script src="https://code.jquery.com/jquery-3.6.1.js" integrity="sha256-3zlB5s2uwoUzrXK3BT7AX3FyvojsraNFxCc2vC/7pNI=" crossorigin="anonymous"></script>
<title>signUp page</title>
</head>
<body>
<h1>signUp page</h1>
<a href="/user/login">로그인</a>
<a href="/">홈</a>
<br />
<br />
<form id="signUpForm">
<input
type="text"
id="id"
name="id"
placeholder="please enter a your id"
/>
<br />
<br />
<input
type="password"
id="pwd"
name="pwd"
placeholder="please enter a your password"
/>
<br />
<br />
<input
type="password"
id="rePwd"
name="rePwd"
placeholder="please enter a your password"
/>
<br />
<br />
<input
type="text"
id="name"
name="name"
placeholder="enter your name sir~"
/>
<br />
<br />
<input type="button" value="signUp" onclick="sendData()" />
</form>
</body>
</html>
자 그럼 해당 index.html파일을 만들었으니 홈이 되는 페이지에서 로그인 페이지와 회원가입 페이지로 이동해봅시다.
router/user.js 라우터 폴더 안에 user.js란 이름으로 라우팅을 하고자 합니다.
express.Router() 매서드를 이용하여 쉽게 라우팅을 할 수 있습니다.
<user.js>
const express = require("express");
const router = express.Router();
// 회원 가입된 사람
let userData = [{
id: 'kane',
pwd: '10',
}];
// http get
// http://localhost:7777/user/login => 로그인 페이지를 보여주고,
router.get("/login", (req, res, next) => {
res.render("login.html");
});
// http://localhost:7777/user/signUp => 로그인 등록 페이지를 보여주고,
router.get("/signUp", (req, res, next) => {
res.render("signUp.html");
});
module.exports = router;
이렇게 매서드를 router에 담아서 router.get을 이용해서 라우팅이 가능합니다.
물론 따로 router폴더를 만들지 않고 app.js에서 구현해도 됩니다. 하지만 복잡하자나요 ^^;;
그리고 로그인, 회원 가입 태그를 클릭하면
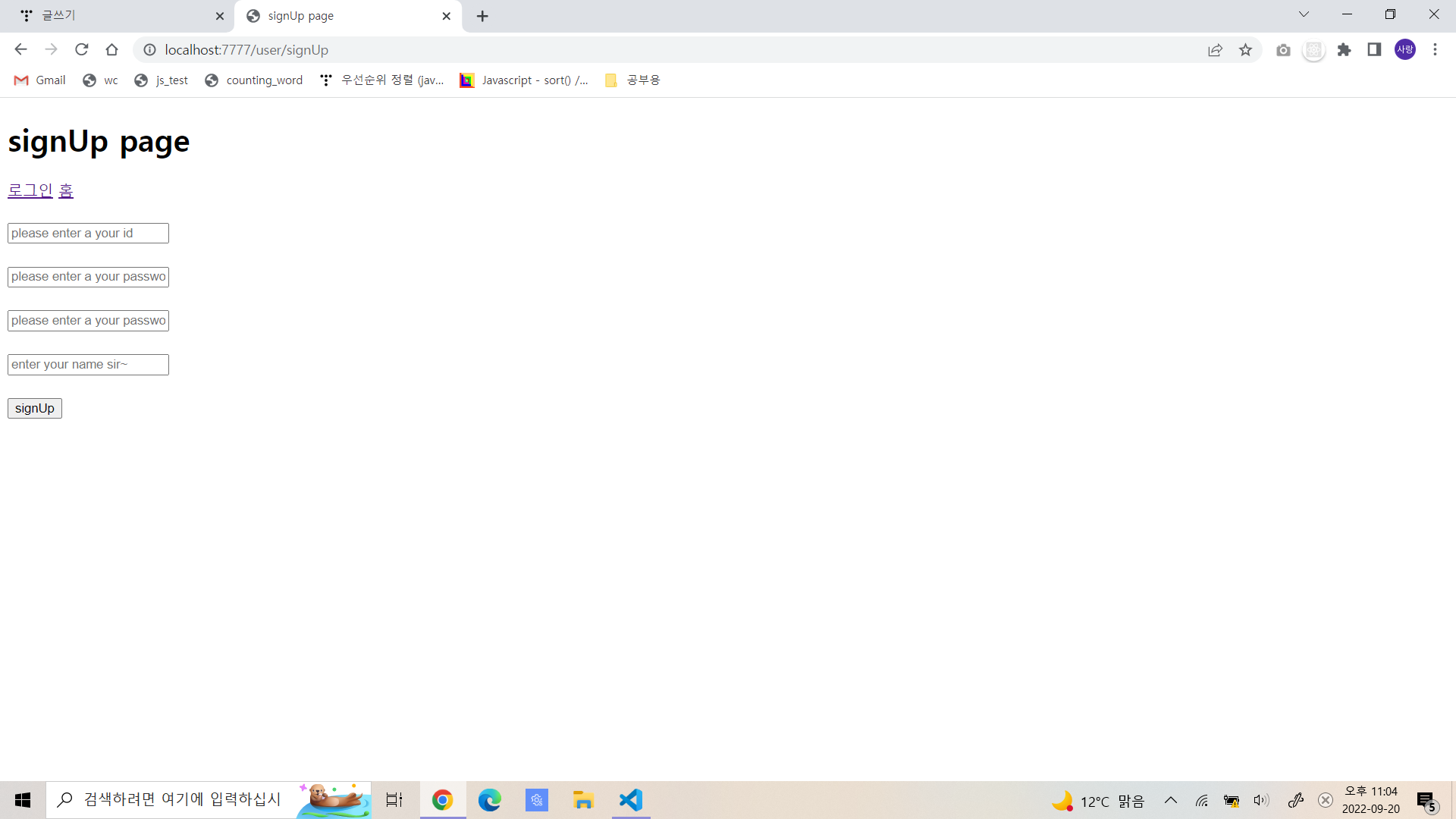
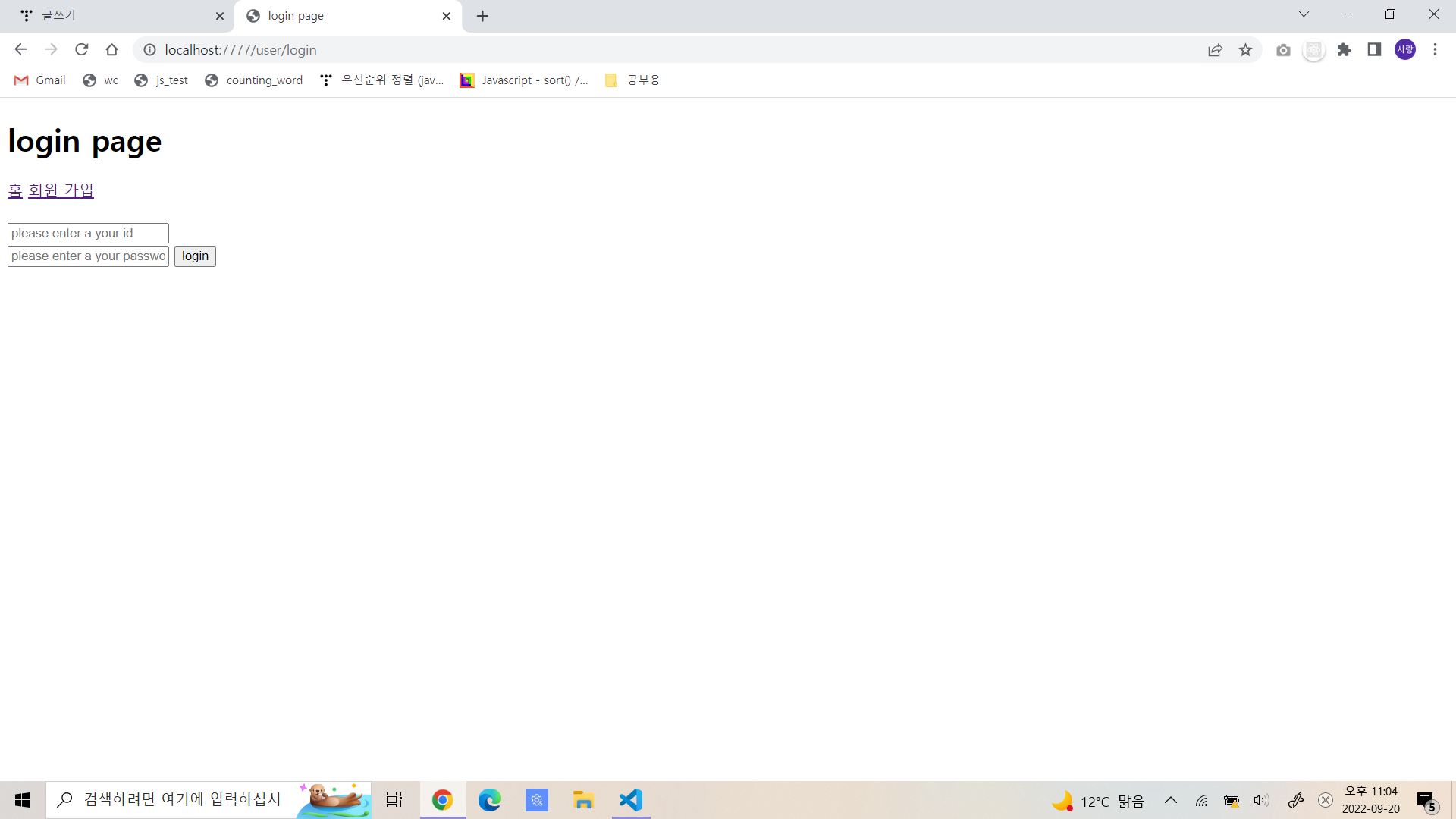
짜잔~
로그인 페이지와 회원 가입페이지가 보이네요.
후기
정상적으로 작동하는 것을 볼 수 있습니다.
다음 포스팅에는 ajax를 이용해서 로그인과 회원 가입에 데이터를 입력하고 전송하는걸
구현해보고자 합니다.
'웹 개념' 카테고리의 다른 글
서버와 웹단을 직접 구현하여 게시판 사이트 만들기 - (1) (2) | 2022.09.25 |
---|---|
express.js 와 ajax를 이용한 라우팅과 비동기 통신 구현(2) (1) | 2022.09.21 |
CSS - "&", ":", cursor은 멀 의미하는 걸까? (0) | 2022.09.03 |
HTML id 와 class 차이? (0) | 2022.09.02 |
css flex? (0) | 2022.09.02 |